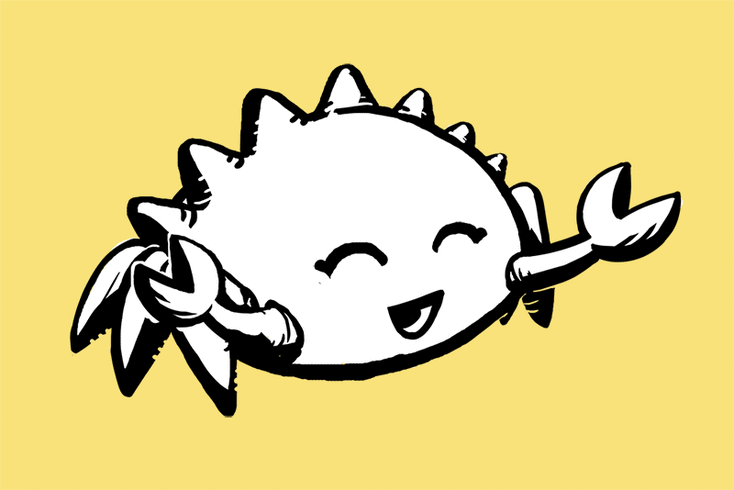
3 Powerful Rust Tips That Will Save Your Development Costs
Discover how Rust's ownership model, concurrent programming, and type system can significantly reduce development costs and improve application reliability. Learn practical tips for memory safety, parallel processing, and dependency management.
Three Rust Tips to Help You Save Money
Rust is a rapidly developing programming language that excels in many aspects including error handling, documentation, and community support. This article will explore how Rust can help reduce costs in the long run through its key features and design principles.
Tip 1: Adopt Rust's Ownership Model to Ensure Memory Security
Rust has a great concept of ownership model that makes memory management and garbage collection very simple. It ensures memory security without the need for a garbage collector, thereby reducing runtime overhead. Bugs such as null pointer dereference, dangling reference, and data contention can be caught at compile time. This greatly reduces debugging and maintenance costs, as memory related errors are one of the most challenging (and expensive) errors in production environments, preventing application crashes and downtime (which can result in revenue loss, customer dissatisfaction, or SLA fines).
For example, consider a service that processes millions of transactions per day. It has the following logic written in C++:
int arr[10];
int service(int i) {
...
arr[i] = 0;
return arr[i];
}
Here, variable i
is input by the user. If i
has a value of 12, the function will run without throwing any errors and return 0 (yes, I was also surprised when I actually tried it). However, this may lead to memory leaks and potential security issues.
Rust cleverly solves this problem by throwing an error indicating that index 12 cannot be updated or accessed. And that's not all. Rust also has a concept of lifetime, which determines the amount of time a variable is available in memory. It can be seen as an enhanced version of the variable scope.
The best thing is that Rust does not sacrifice performance while implementing these features, avoiding the pitfalls of manual memory management and garbage collection.
Tip 2: Writing Concurrent Rust Code
Let's discuss this issue through an example. Consider the following code snippets written in JavaScript:
const numbers = [
....
];
let output = [];
for (const number of numbers) {
// A high computational function
const out = await compute(number)
output.push(out);
}
Here, we called a computationally intensive asynchronous function. However, this function will run on the main thread for each iteration. Therefore, if one iteration takes 10 seconds and there are 100 iterations, the loop will run for 1000 seconds, which is 16.67 minutes.
Let's see how Rust can help solve this problem. Rust allows us to run tasks on different threads. Therefore, if we want to run the same code in Rust, we can do this:
let numbers = vec![
....
];
let mut output = Vec::new();
for number in numbers {
// Await the computation
let out = compute(number).await;
output.push(out);
}
A better approach would be:
let numbers = vec![
....
];
let futures = numbers.into_iter().map(compute);
let output: Vec<i32> = join_all(futures).await;
In the first scenario, each number is processed sequentially, taking O(n) time. In the second scenario, all computation calls are created as futures and run concurrently using join_all
, reducing the time complexity to O(1) (assuming no additional overhead).
This parallel processing capability in Rust is more efficient than Node.js workers as it uses shared memory while enforcing security at compile time, rather than relying on independent processes.
Tip 3: Implementing Strong Typed Code with Types
Rust provides a powerful type system that helps catch errors at compile time. For example:
// u32
let x1 = 1;
// Vec<u32>
let x2: Vec<u32> = vec![];
Similar to TypeScript, Rust can either infer types automatically or allow explicit type annotations. For handling null values, Rust uses the Option<T>
enum:
enum Option<T> {
None,
Some(T),
}
This approach, combined with Rust's pattern matching, provides a more elegant solution than traditional null checks.
Extra Tip: Utilize Rust's Crate System
Rust's package management system, Cargo, provides several advantages:
- Automatic dependency locking with
Cargo.lock
- Efficient dependency caching
- Compile-time security checks
- Type safety verification for all dependencies
- Reproducible builds across systems
These features help ensure consistent development environments and reduce "works only on my machine" issues.
Related Posts
GitHub Daily Recommendation 2025-02-06
🚀 GitHub Daily Recommendations Alert! 🌟 Today's spotlight is on AI Artificial Intelligence projects. Dive into cutting-edge code, discover top-notch tools, and elevate your AI skills! 🤖💡 #GitHub #AI #OpenSourceProjects
Product Hunt Daily Recommendation 2025-02-06
Discover the latest Product Hunt daily recommendations for today! Dive into top AI tools, SaaS products, and mobile apps. Explore innovative tech and stay ahead in the world of startups. Join us for a daily dose of cutting-edge innovation!
GitHub Daily Recommendation 2025-02-05
🚀 GitHub Daily Recommendations Alert! 🌟 Dive into today's top-notch open-source projects, including cutting-edge AI, stunning Web Frontend, and powerful Cloud Computing solutions. Enhance your tech toolkit and stay ahead with our 🎯 curated picks! 📚✨