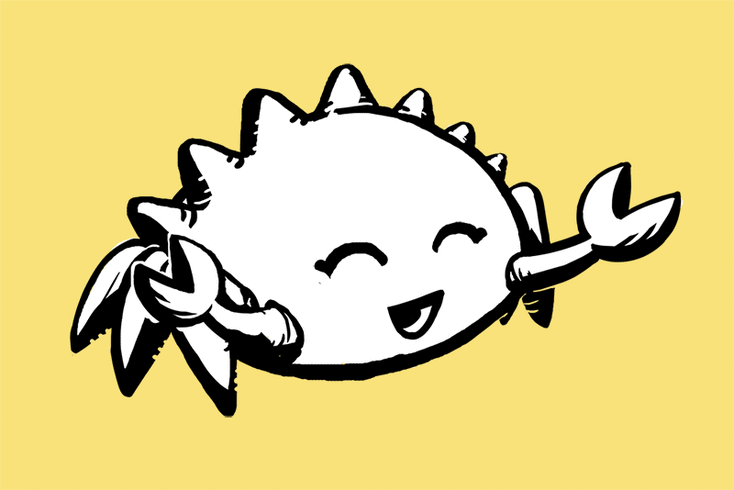
Rust Async Runtime Battle: Glommio vs Monoio Performance Showdown
Deep dive into Rust's async runtimes - comparing Glommio and Monoio's performance in high-concurrency scenarios. Learn which runtime excels in I/O operations, resource efficiency, and specific use cases to make the best choice for your next project.
Rust Asynchronous Runtime: Comparing Glommio and Monoio in High-Concurrency Environments
Introduction
In modern server development, choosing the appropriate asynchronous runtime is crucial for performance and resource consumption. Rust, as an emerging system programming language, is renowned for its powerful memory safety and high performance. Rust's asynchronous programming model, combined with tokio
, Glommio
, and Monoio
libraries, can achieve optimal performance in different scenarios. This article explores in-depth which scenarios Glommio performs best in, and whether Monoio or Tokio is more suitable for high-concurrency environments in practical applications.
1. Optimal Scenarios for Glommio
Glommio
is an asynchronous runtime based on Linux io_uring, particularly suitable for high-performance I/O-intensive applications. Here are scenarios where Glommio performs best:
1.1 High-Performance I/O-Intensive Applications
Glommio
provides extremely high I/O performance by utilizing Linux io_uring technology. It's especially suitable for applications requiring numerous file read/write and network I/O operations.
use glommio::LocalExecutor;
use glommio::io::{DmaFile, DmaStreamWriter};
use std::time::Duration;
fn main() {
let ex = LocalExecutor::default();
ex.run(async {
let file = DmaFile::create("example.txt").await.unwrap();
let mut writer = DmaStreamWriter::new(file);
writer.write_all(b"Hello, world!").await.unwrap();
});
}
1.2 Low-Latency Applications
Due to io_uring characteristics, Glommio
can significantly reduce I/O operation latency, making it particularly suitable for applications with extremely high latency requirements, such as real-time data processing and high-frequency trading.
use glommio::LocalExecutor;
use glommio::net::TcpListener;
use glommio::io::{AsyncReadExt, AsyncWriteExt};
fn main() {
let ex = LocalExecutor::default();
ex.run(async {
let listener = TcpListener::bind("127.0.0.1:8080").await.unwrap();
loop {
let (mut socket, _) = listener.accept().await.unwrap();
glommio::spawn(async move {
let mut buf = [0; 1024];
let n = socket.read(&mut buf).await.unwrap();
println!("GOT {:?}", &buf[..n]);
socket.write_all(&buf[..n]).await.unwrap();
});
}
});
}
2. Monoio vs. Tokio in High-Concurrency Environments
Choosing the appropriate asynchronous runtime is crucial in high-concurrency environments. Let's compare Monoio
and Tokio
:
2.1 Monoio
Monoio
is an io_uring-based asynchronous runtime, particularly suitable for high-performance network applications.
Advantages:
- High-Performance Networking: Provides extremely high network performance using io_uring technology
- Low Resource Consumption: Optimized thread management and resource allocation
use monoio::net::TcpListener;
use monoio::io::{AsyncReadExt, AsyncWriteExt};
#[monoio::main]
async fn main() {
let listener = TcpListener::bind("127.0.0.1:8080").await.unwrap();
loop {
let (mut socket, _) = listener.accept().await.unwrap();
monoio::spawn(async move {
let mut buf = [0; 1024];
let n = socket.read(&mut buf).await.unwrap();
println!("GOT {:?}", &buf[..n]);
socket.write_all(&buf[..n]).await.unwrap();
});
}
}
2.2 Tokio
Tokio
is one of Rust's most popular asynchronous runtimes, widely used in various high-concurrency scenarios.
Advantages:
- Mature and Stable: Extensively tested and proven in production environments
- Rich Ecosystem: Provides numerous tools and libraries for building complex applications
use tokio::net::TcpListener;
use tokio::io::{AsyncReadExt, AsyncWriteExt};
#[tokio::main]
async fn main() {
let listener = TcpListener::bind("127.0.0.1:8080").await.unwrap();
loop {
let (mut socket, _) = listener.accept().await.unwrap();
tokio::spawn(async move {
let mut buf = [0; 1024];
let n = socket.read(&mut buf).await.unwrap();
println!("GOT {:?}", &buf[..n]);
socket.write_all(&buf[..n]).await.unwrap();
});
}
}
2.3 Selection Guidelines
Choose based on your specific requirements:
-
Choose Monoio for:
- Extremely high network performance requirements
- Low resource consumption needs
- High-latency sensitive applications
-
Choose Tokio for:
- Production-ready stability
- Complex business logic handling
- Need for extensive ecosystem support
Conclusion
Each runtime has its strengths in different scenarios:
- Glommio excels in I/O-intensive and low-latency applications
- Monoio shines in high-performance networking with low resource overhead
- Tokio provides stability and ecosystem maturity
The choice between these runtimes should be based on your specific use case, performance requirements, and development constraints. Through proper selection and optimization, Rust's async runtimes can deliver optimal performance across different scenarios, bringing higher performance and reliability to server applications.
Related Posts
GitHub Daily Recommendation 2025-02-06
🚀 GitHub Daily Recommendations Alert! 🌟 Today's spotlight is on AI Artificial Intelligence projects. Dive into cutting-edge code, discover top-notch tools, and elevate your AI skills! 🤖💡 #GitHub #AI #OpenSourceProjects
Product Hunt Daily Recommendation 2025-02-06
Discover the latest Product Hunt daily recommendations for today! Dive into top AI tools, SaaS products, and mobile apps. Explore innovative tech and stay ahead in the world of startups. Join us for a daily dose of cutting-edge innovation!
GitHub Daily Recommendation 2025-02-05
🚀 GitHub Daily Recommendations Alert! 🌟 Dive into today's top-notch open-source projects, including cutting-edge AI, stunning Web Frontend, and powerful Cloud Computing solutions. Enhance your tech toolkit and stay ahead with our 🎯 curated picks! 📚✨