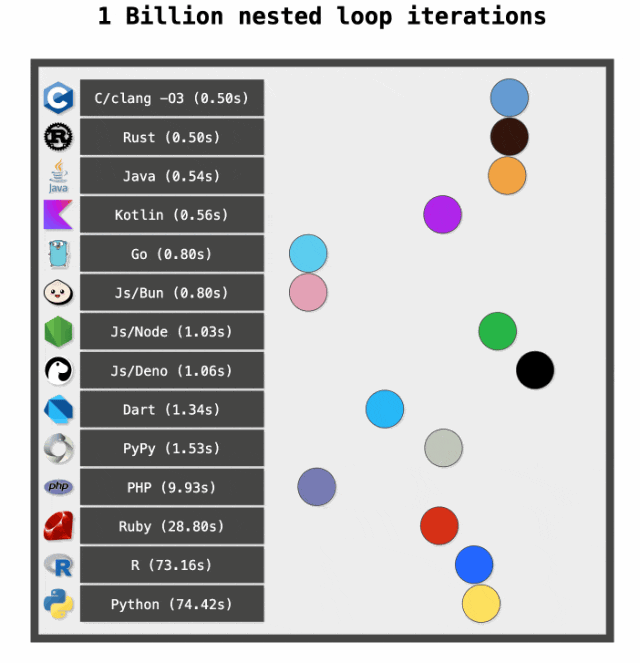
King of Performance: The Fastest Programming Language
A comprehensive comparison of programming language performance through billion-level loop iterations, featuring C, Rust, Zig, and Go.
King of Performance: The Fastest Programming Language
Ha, once there is such a comparison, it will be hyped up. See you in the comment section.
Ben Dicken (@BenjDicken) conducted a test to execute a double loop, 10000 * 100000=1 billion loops, to see which programming language is faster.
The code implementations for various languages can be found in the project code repository: 1 Billion nested loop iterations.
C Code
#include "stdio.h"
#include "stdlib.h"
#include "stdint.h"
int main(int argc, char** argv) {
int u = atoi(argv[1]); // Get an input number from the command line
int r = rand() % 10000; // Get a random integer 0 <= r < 10k
int32_t a[10000] = {0}; // Array of 10k elements initialized to 0
for (int i = 0; i < 10000; i++) { // 10k outer loop iterations
for (int j = 0; j < 100000; j++) { // 100k inner loop iterations, per outer loop iteration
a[i] = a[i] + j%u; // Simple sum
}
a[i] += r; // Add a random value to each element in array
}
printf("%d\n", a[r]); // Print out a single element from the array
}
Rust Code
use rand::Rng;
fn main() {
let n: usize = std::env::args() // Get an input number from the command line
.nth(1)
.unwrap()
.parse()
.unwrap();
let r: usize = rand::thread_rng() // Get a random number 0 <= r < 10k
.gen_range(0..10000);
let mut a = [0; 10000]; // Array of 10k elements initialized to 0
for i in 0..10000 { // 10k outer loop iterations
for j in 0..100000 { // 100k inner loop iterations
a[i] = a[i] + j % n; // Simple sum
}
a[i] += r; // Add a random value to each element in array
}
println!("{}", a[r]); // Print out a single element from the array
}
Zig Code
const std = @import("std");
const rand = std.crypto.random;
const stdout = std.io.getStdOut().writer();
pub fn main() !void {
// Get an input number from the command line
var args = std.process.args();
_ = args.next() orelse unreachable; // skip first, which is program name
const arg = args.next() orelse unreachable;
const u = try std.fmt.parseInt(usize, arg, 10);
// Get a random number 0 <= r < 10k
const r = rand.intRangeAtMost(usize, 0, 10000);
// Array of 10k elements initialized to 0
var a: [10000]usize = undefined;
@memset(&a, 0);
// 10k outer loop iterations
for (0..10000) |i| {
// 100k inner loop iterations, per outer loop iteration
for (0..100000) |j| {
a[i] += j % u; // Simple sum
}
a[i] += r; // Add a random value to each element in array
}
try stdout.print("{d}\n", .{a[r]}); // Print out a single element from the array
}
Go Code
package main
import (
"fmt"
"math/rand"
"strconv"
"os"
)
func main() {
input, e := strconv.Atoi(os.Args[1]) // Get an input number from the command line
if e != nil { panic(e) }
u := int(input)
r := int(rand.Intn(10000)) // Get a random number 0 <= r < 10k
var a[10000]int // Array of 10k elements initialized to 0
for i := 0; i < 10000; i++ { // 10k outer loop iterations
for j := 0; j < 100000; j++ { // 100k inner loop iterations, per outer loop iteration
a[i] = a[i] + j%u // Simple sum
}
a[i] += r // Add a random value to each element in array
}
fmt.Println(a[r]) // Print out a single element from the array
}
The test results are as follows (the author tested each language several times and selected the fastest one for each language). The author also used animation to demonstrate the speed of each language very well. The faster the execution, the shorter the execution time, and the faster the ball moves:
My own testing also includes C, Zip, and Rust, which belong to the first tier.
The author also provided testing of the Fibonacci function, so overall, these two scenarios were mainly tested.
Which programming language do you support, or are you staying out of the matter?
References
- Billion nested loop iterations](https://github.com/bddicken/languages)
Related Posts
GitHub Daily Recommendation 2025-02-06
🚀 GitHub Daily Recommendations Alert! 🌟 Today's spotlight is on AI Artificial Intelligence projects. Dive into cutting-edge code, discover top-notch tools, and elevate your AI skills! 🤖💡 #GitHub #AI #OpenSourceProjects
Product Hunt Daily Recommendation 2025-02-06
Discover the latest Product Hunt daily recommendations for today! Dive into top AI tools, SaaS products, and mobile apps. Explore innovative tech and stay ahead in the world of startups. Join us for a daily dose of cutting-edge innovation!
GitHub Daily Recommendation 2025-02-05
🚀 GitHub Daily Recommendations Alert! 🌟 Dive into today's top-notch open-source projects, including cutting-edge AI, stunning Web Frontend, and powerful Cloud Computing solutions. Enhance your tech toolkit and stay ahead with our 🎯 curated picks! 📚✨